An Object is a box containing information and functions.
Object-Oriented Programming is a way of writing code that allows you to create different objects from a common object. Also, Object-Oriented Programming has been around since the 70s and It is a style of programming that is very common in languages such as C#, Python, Ruby, and Java.
JavaScript Object
let tim = {
name: "Tim",
country: "USA",
speak(language) {
if (language.toLowerCase() == "english") {
return true;
}
return false;
}
}
console.log(tim.country);
console.log(tim.speak("english"));
USA
true
the tim object have some Object-Oriented Principles.
tim object contains name, country and a speak function.
In comes to Object-Oriented Programming there are two main types there is class-based programming languages and prototype-based programming languages. JavaScript is a prototype-based programming language.
Object-Oriented Programming: Organize your code.
Basic
const tim = {
name: "Tim",
country: "USA",
language: "english",
speak() {
return tim.name + " can speak " + tim.language
}
}
const mike = {
name: "Mike",
country: "Spain",
language: ["english", "spanish"],
speak() {
return mike.name + " can speak " + mike.language.toString();
}
}
console.log(tim.speak())
console.log(mike.speak())
Tim can speak english
Mike can speak english,spanish
Object-Oriented Programming what we have something called Encapsulation. tim
and mike
are containers. That is good. However, if we have more people we need more and more objects. We should have to copy and paste the same code over and over. That is not our code very dry.
Sheared Object.
function createPeople(name, country, language){
return {
name: name,
country: country,
language: language,
speak(){
return name + " can speak " + language;
}
}
}
const tim = createPeople("Tim", "USA", "english")
const mike = createPeople("Mike", "Spain", ["english", "spanish"])
console.log(tim.speak());
console.log(mike.speak());
Tim can speak english
Mike can speak english,spanish
We have created a factory function that is a function that creates an object for us. We avoided repetitive code. However, Still, there is a problem. What if we had a thousand people which means a thousand people require our memory to store the same data. Things like name, country, and language are different but speak() function is pretty generic. So what we can do is
Shared Object with Share Function
function createPeople(name, country, language) {
return {
name: name,
country: country,
language: language,
}
}
const speakFunction = {
speak() {
return this.name + " can speak " + this.language;
}
}
const tim = createPeople("Tim", "USA", "english")
tim.speak = speakFunction.speak
const mike = createPeople("Mike", "Spain", ["english", "spanish"])
mike.speak = speakFunction.speak
console.log(tim.speak());
console.log(mike.speak());
Tim can speak english
Mike can speak english,spanish
JavaScript has a prototypal Inheritance. We can use that to our advantage to maybe improve with sharing functionality across different objects.
BUT we have shared functionality, it is still a lot of manual work.
Object.create()
function createPeople(name, country, language) {
let newPeople = Object.create(speakFunction);
newPeople.name = name;
newPeople.country = country;
newPeople.language = language;
return newPeople;
}
const speakFunction = {
speak() {
return this.name + " can speak " + this.language;
}
}
const tim = createPeople("Tim", "USA", "english")
const mike = createPeople("Mike", "Spain", ["english", "Spanish"])
console.log(tim);
console.log(tim.speak());
console.log(mike.speak());
console.log(tim.__proto__);
console.log(tim.__proto__.__proto__);
{ name: 'Tim', country: 'USA', language: 'english' }
Tim can speak english
Mike can speak english,Spanish
{ speak: [Function: speak] }
{}
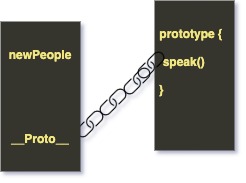
What is Object.create()?
it creates a link between the speakFunction and newPeople. This is a prototypal Inheritance. However, some people do not like Object.create() style.
If you do not like this style, you can consider using class keyword in Java.
Most of the JavaScript community does not use Object.create() as much as class syntax.